I’ve started to feel that the codebase for this game is getting to a point where the volume of code is having an effect on the complexity. It felt to me that every time I wanted to make a small change, regardless of how elegantly I felt I was doing so, I was doing more work than necessary to get it implemented.
Curious to back up my feelings, I imported my repository into a webapp called Gitential that helps visualize various datapoints around a project on Github. The app is fantastic, but what I found interesting was a certain graph that showed that, while the codebase was increasing in complexity initially, the complexity was trending downward over time as the volume of code increased.
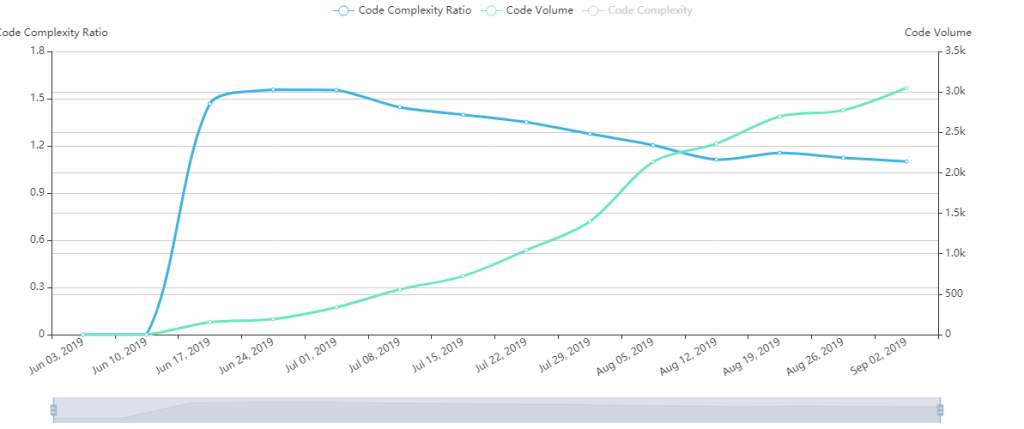
The app maintains that the complexity increases as new code is added to the code base, but decreases as refactoring is done. I make sure to refactor as much as I can, leaving notes in places where a refactoring would be more troublesome.
An Improved Cast
One such refactoring was how we were handling Casts
. Casts
are how I am handling Entity
to Entity
interaction in the game world. Basically, an Entity
will send out a Cast
to query the map at a certain set of coordinates. If it finds something, it’ll trigger an interaction: a message will be displayed, a chest will open up, a warp tile will send you to another part of the map:
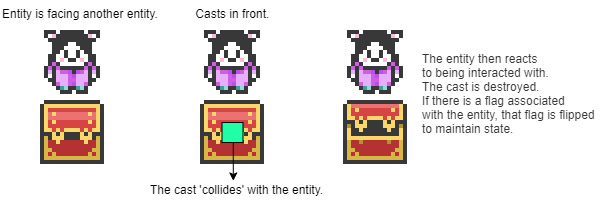
A reference to a group of Casts
that lived in the Scene
object was passed around to every Entity
object that exists on the Scene
via their constructors. When an Entity
“casted”, it added its Cast
to this object directly. This tight coupling was very bad for complexity. It meant that my classes knew too much about other classes.
So I tore that Cast
group out of the constructors of all of my Entities
, and instead had the Entity
emit a Cast
event
that the Scene
was listening for. That way the implementation was decoupled.
I also gave the Cast
a little bit more. I made an enum for the type of cast being casted– I wanted to differentiate between a cast that was sent out (up, down, left or right) from the Entity
and a cast being sent below it to query underfoot. I named them reach
and pressure
Casts
, respectively.
I also added an event to the Cast
that will emit riiiight before it fizzles out and dies forever, sending back valuable information to the caster about the Entity
that it found. This allows Entities
to query other Entities
.
Boss Monsters
One of my epic milestones was to create a a special type of battle for boss monsters. These would be battles that were triggered by NPC
s on the world map, and upon winning would flip a Flag
or series of Flags
in the world.
I designed some bosses, wrote some dialog for them, and placed them on the world map. I wanted the boss’s death to trigger a key item to spawn in the space that it died on.
Cosmoknight Laundromancer Wanmonster
I wrote a method that would iterate through every Entity
on the map and show or hide it based on whether the flag was flipped. When hidden, the Entity
would query underfoot using a Cast
before disappearing and turning the tile’s collision off.
The result was an experience where the player triggers a battle by talking to the boss monster, wins the battle, the boss Sprite is gone and in its place was an item to collect.
Loot



I created a new class of Item
that is specifically made for selling: Loot
. I designed things like jewels and precious gems and set some monsters to have a very small chance of dropping them.
When the store is implemented later, the player will be able to sell them for large amounts of gold.
Locked Doors and Chests

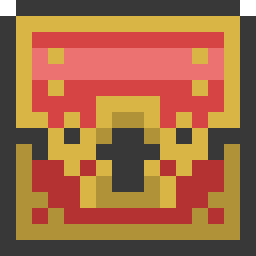
I also introduced a new type of Entity
: locked doors and locked chests. These can be opened by collecting silver and gold keys respectively. The chests can contain great treasure like the above-mentioned Loot
or KeyItems
, and the locked doors can gate player progress.
These can be really useful tools for creating puzzles and guiding the player through the game.
Moving on, the next big step is adding some more features to the battle system. I’m in the middle of scoping this out and designing some of the new elements, but I look forward to sharing my progress there in the coming weeks!