Over the course of the past year, my son has been having difficulty regulating his big emotions. Part of this, I realize, is being a three year old. It’s kind of unavoidable that a meltdown will happen. His brain is still developing. I realized that I wanted to do as much as I could to meet him halfway, help give him an environment where he can be free to express his strong emotions.
It started out with simply acknowledging them, and making sure he felt heard. It evolved into trying to draw them, or express them physically by punching pillows and stuffed animals. Eventually, Lo bought a series of posters we have hung in the kitchen area where he can easily point and identify with what he’s feeling. There are red, green, blue and yellow areas representing anger, happiness, sadness and anxiety/silliness respectfully.
I wanted to take that a step further, by building a small app that allows him to identify his emotions in a visually pleasing and tactile way. I set out to make a digital form of the posters we were using to great effect in the kitchen.
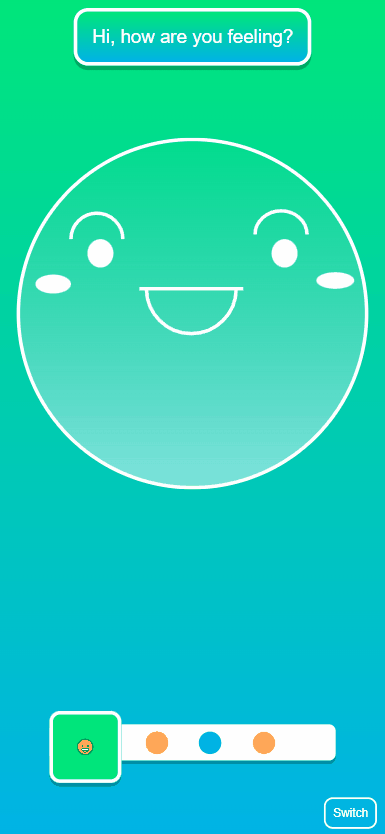
Aris (and Ander, as it turns out!) can slide the slider left or right to choose an emotion, select it, and engage in a small activity that can help redirect and soothe stronger emotions.
What I focused on chiefly for this app is to not overload the user experience with bloat. The app is designed for small children who are in the midst of the most overwhelming moments of their life. It needed to have a super-abbreviated, super-simple user experience that gets to the point and immediately helps soothe big emotions. My goal is to develop dead-simple “mini-games” or “activities” associate with each mental state to help regulate and bring their minds back to a place where they can make better decisions.
I’ll keep updating as the app continues to develop. In the meantime, feel free to try it out at the development endpoint, here: EmotionApp